Weeks 5 and 6 were mostly spent on successfully informing the PDE whenever Shdr's code was compiled successfully after some changes were made by the user. This came out to be more challenging as expected.
For this I looked at the Shdr code files in more detail to find out where the check for successful recompilation of shader was taking place. This was found out to be in the updateShader function in a file called App.js, which was also the main file that controlled all the other processes of Shdr.
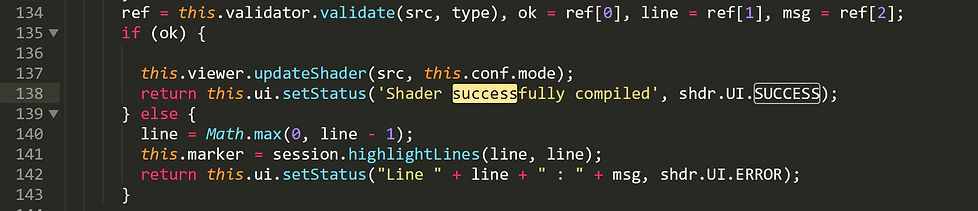
The goal was to pass the value of the variable 'ok' to the PDE which after assessing the value would recompile its own default shader. However sending this value proved to be a more challenging task than expected. I first tried to make the 'ok' variable global so that it could be accessed by any javascript file(index.js in my case) in the project as Javascript allows that. I tried to access the value in index.js file so that it could be sent to the PDE from there using IPC. However the value could not be accessed as I found out later that index.js was on a different thread than that of App.js. In other words, electron is composed of two different processes, the main and the renderer process.
"Each of these processes run concurrently to each other. The most important thing to remember here is that processes’ memory and resources are isolated from each other." link
The only way to make the processes communicate was through IPC using electron's IPC module which comprises ipcMain (required on server side) and ipcRenderer (required on client side). The server here was index.js while the client was editor.html. I added a button in editor.htm that would send a message to index.js whenever it was pressed.
Note: The client could also be a javascript file on the renderer side but that didn't work for me(compiler couldn't find/access the file maybe because Shdr had to be rebuild) so I chose the html file.
Index.js
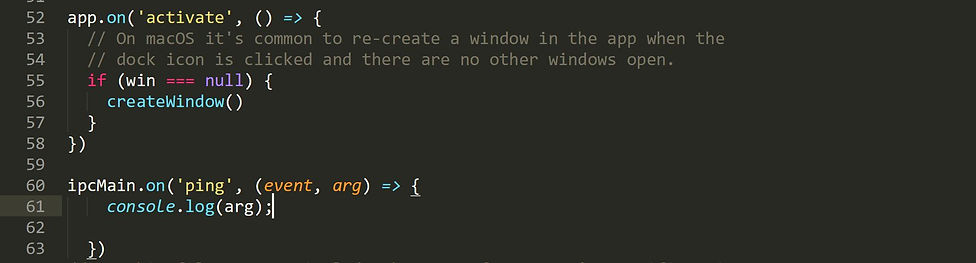
Editor.html
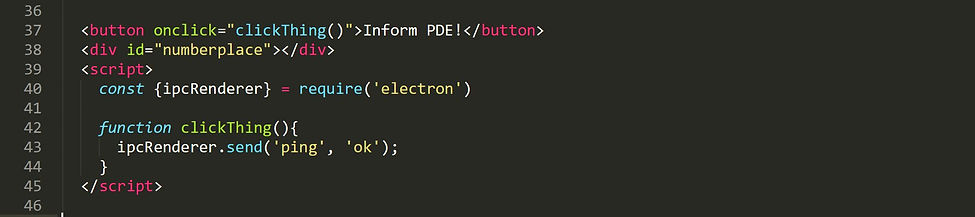
Now whenever I ran both the applications, the PDE first and then the Hello Tool followed by starting Shdr through electron(I was starting Shdr directly from within the PDE before but that made it difficult to debug errors in Shdr so I moved back to starting Shdr from the command window) a button would appear on Shdr. Whenever this button was pressed a 'ping' message would be printed on the console (command prompt).
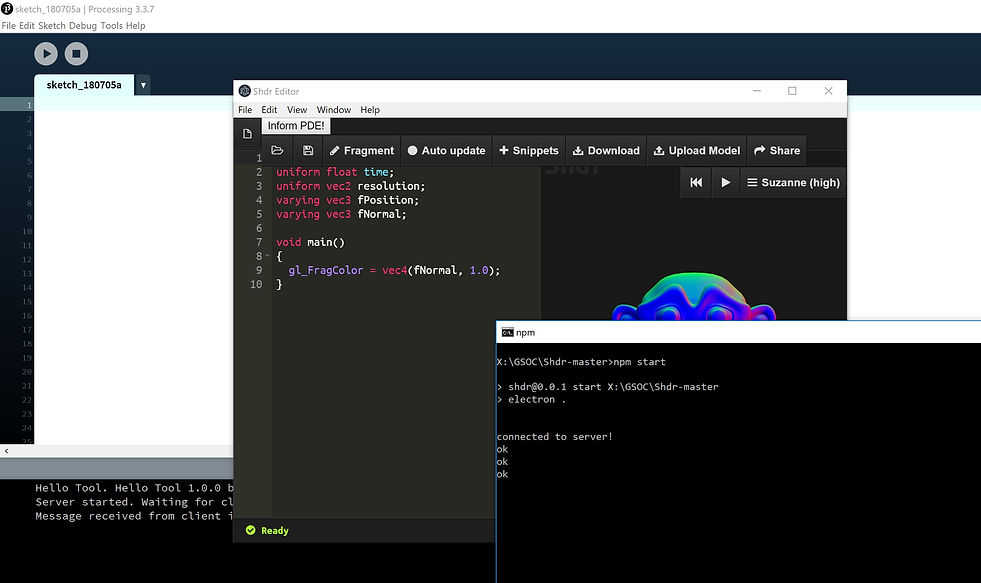
Now the same message needed to be sent to the PDE. For that I had to move ipcMain.on() inside the client.on function otherwise the client.on() wouldn't function i.e it wouldn't send the message to the PDE (found through trial and error). The next step was sending the actual value of 'ok' instead of the string. Here one thing to note was that 'ok' was a local variable declared inside the scope of a function in App.js i.e it couldn't be accessed anywhere else outside the function let alone the editor.html file. In order to make the value public and accessible I declared 'ok' right at the top of the App.js file so that it became a global variable. Now it could be accessed inside the editor.html file directly as shown below.

And this is how it looked when it was tested,
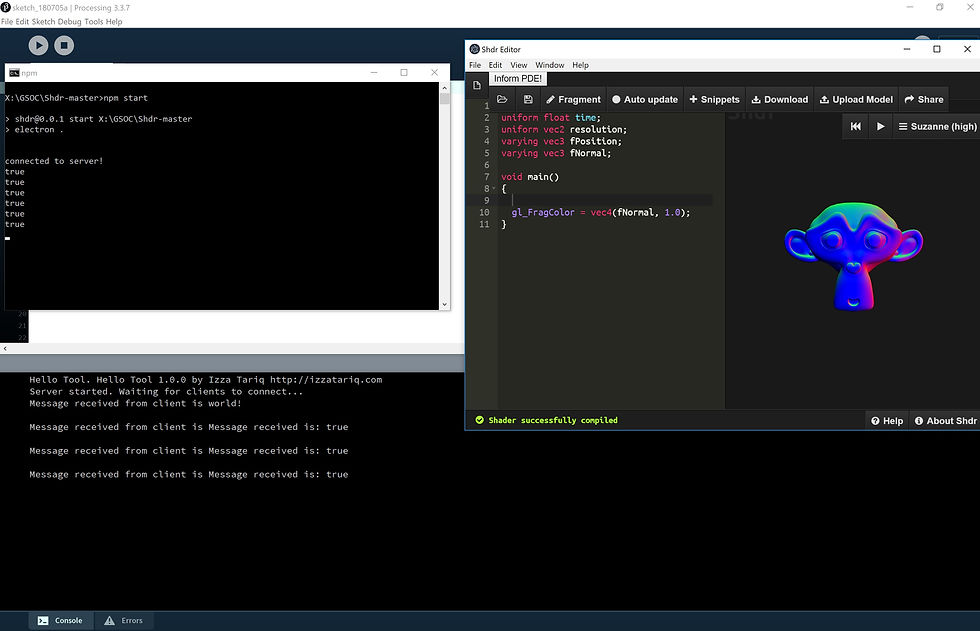